In this article, we will talk about the differences between var, let, and const. After reading this article you will have a clear idea about when to choose one over the other.
ES6, also know as ECMAScript 6, which introduced in 2015, added two new ways to define variables in JavaScript. Let
, and const
are those new keywords that we can use in JS. Before that, you could only use var
to define variables in JavaScript.
So, what’s the big idea? Why are three different ways to define variables? Which one to choose? Before that, Let’s talk about what is scope means in JavaScript. After that move into the var, let, and const difference in JavaScript and which to use?
Table of Contents
Types of scopes in JavaScript
There are four types of scopes in JavaScript.
- Global scope – visible for every part of your program
- Block scope – visible within a block
- Function scope – visible within a function
- Module – visible within a module
Note: what is a block?
A scope is an area within if control statement, switch conditions,
for
andwhile
loops. Generally speaking, whenever you see curly brackets ( {}), it is a block.
Var
Var
is the function scope. Their visibility is limited to the function. When you try to use it outside the function scope, you will get an error.
function sum() {
var total = 5 + 5;
// output is 5
console.log("total is: " + total);
}
// Uncaught ReferenceError: total is not defined
console.log("total is: " + total);
Here, I have opened the script file I have written in Google Chrome developer tools. You can see that red squiggly line indicating there is something wrong.
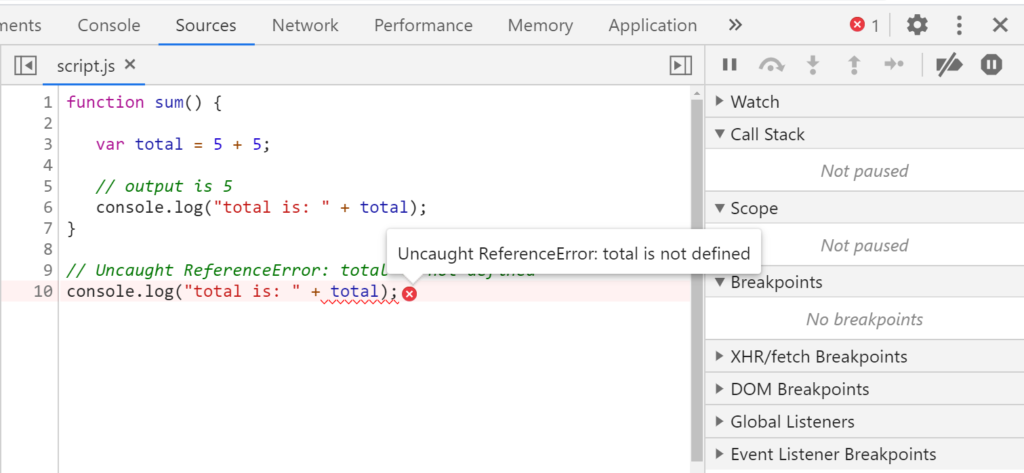
When you are declaring a variable, you can redeclare it, reassign any value as well. Optionally you can omit the value when declaring.
function reAssignAndReDeclare() {
var num = 3;
num = 5; // reassign
var num = 88; // redeclare
console.log(num);
}
what’s the output of above code snippet ? let’s call this function in Google Chrome developer console.
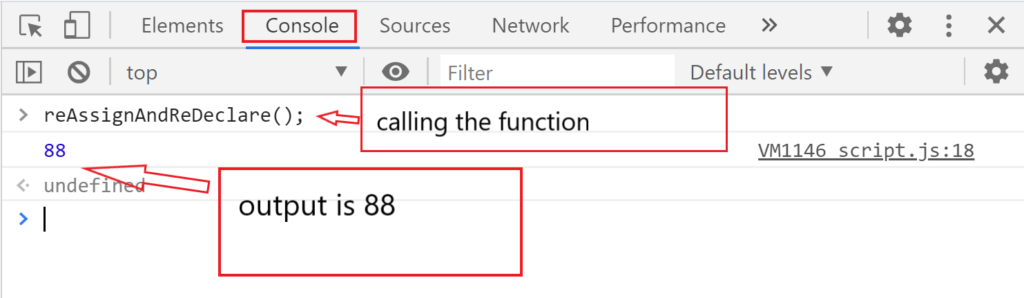
Another critical point is global variables added to the window
object. Let’s say I declared a variable with var
outside a function. Now, Javascript treats this as a global variable and attaches this variable to the window
object.
Since there is only one instance of the window
object, attaching our global variables to that going to have a problem in our code. So, it isn’t good practice.
Let’s say you are using any third-party library with the same variable names as you defined before (in the global context). Then you are running into risk of using your variable values since it can be overridden.
Let’s summarize key points related to the var
keyword.
- Scoped to “current execution context,” also known as “a variable’s enclosing function or the global scope. (function scoped)
Var
is not blocked scope variable- Can be re-assigned whenever
- Initializing the value is optional
- Can be redeclared at any point
- Global variables add to the
window
object
After ES2015 / ES6, we have let
and the const
for declaring variables. Let’s dive into the nuts and bolts of var, let, and const difference in JavaScript and which to use?
Let
As a good practice, we want our variables to scoped within a block of code. If we declare a let
variable within a block scope (if
, for
, while
, etc.), we want it to be accessible within that scope, and inaccessible outside of that block.
Let take an example with for
loop to demonstrate the above point.
// for loop
for (let i = 0; i < 4; i++) {
console.log(i);
}
// Uncaught ReferenceError: i is not defined
console.log(i);
what’s the output of above code snippet ? let’s call this function in Google Chrome developer console.
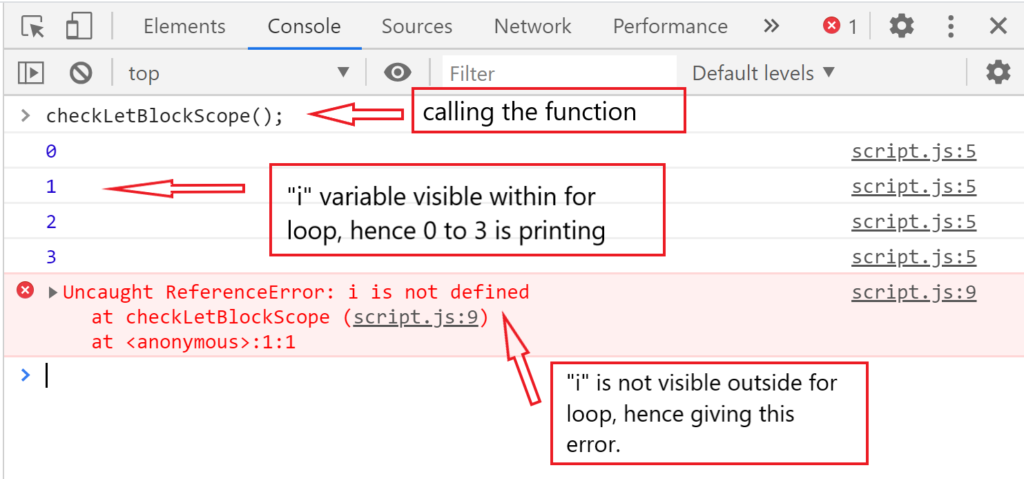
Using the let
variable, you can reassign some value as well. But keep in mind that you cannot redeclare the same variable within the same scope.
function checkLetReDeclareAndRedefine() {
let fruit = "mango";
// reassign is valid
fruit = "orange";
// Uncaught SyntaxError: Identifier 'fruit' has already been declared
let fruit = "banana";
console.log(fruit);
}
// Uncaught ReferenceError: fruit is not defined
console.log(fruit);
here’s the output when you run above code snippet.

Let’s summarize key points related to let
keyword.
- Block scoped
- Does not create a property on the global
window
object - Initializing the value is optional
- Can be reassigned
- It cannot redeclare. (in the same scope)
Const
The keyword const
coming from constant. It’s just an abbreviation. Similar to let
, it’s block scoped. However, you can not re-assigned it.
function constExample() {
const fruit = "orange";
// Uncaught TypeError: Assignment to constant variable.
fruit = "mango";
console.log(fruit);
}
What do you think is the output of this code?
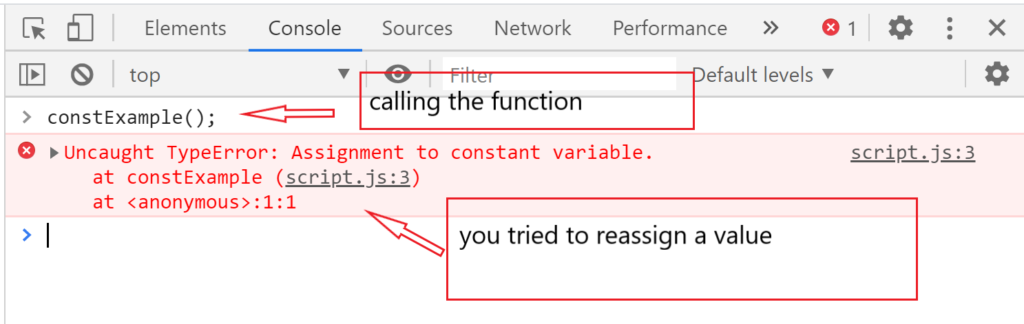
Now, I want to show something related to object creation using const
. When setting a const
variable to an object
, you can modify the object
properties.
function constExampleWithObject() {
const fruit = {
name: "mango",
isRiped: true,
color: "red"
};
// assign value to object property
fruit.isRiped = false;
console.log(fruit.isRiped);
}
let’s see the output of above code snippet.
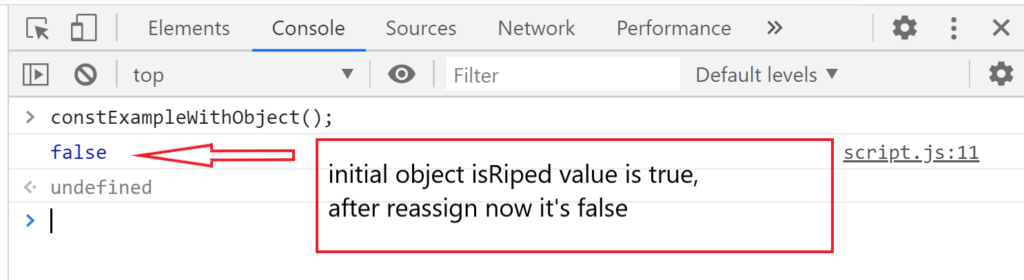
Let’s summarize key points related to the const
keyword in JavaScript.
- Cannot be reassigned
- Not immutable, but variable reference cannot change.
- Block scoped
- Must be initialized with a value
- Does not create a property on the global
window
object - Cannot be redeclared. (in the same scope)
Conclusion
Alright, now you have clear understating about each keyword, Which to use where. Use let
and const
instead of var
for more predictable code in JavaScript. var, let, and const difference in JavaScript and which to use? Now you get the answer you’re looking for.
- Prefer
const
overlet
- Prefer
let
overvar
- You don’t need
var
anymore
Refer this for more information, If you want to read.
Very descriptive post, I loved that a lot. Will there be a part 2?